COBOL Table Processing
COBOL arrays are also known as tables. Array is a collection of individual data items of same type and length. It is a linear data structure that uses to store the identical data repeated multiple times. For example - student marks for all subjects.
Below list of topics we are going to discuss in this chapter -
- Table Declaration
- Subscript
- Index
- SET Statement
- Search
- Search all
Table Declaration -
Tables are declared in the DATA DIVISION. OCCURS clause is used to declare the table in program. It specifies the number that represents how many times the data item is repeated in the table.
01 table-name.
02 variable [PIC data-type(length1)]
OCCURS integer1 TIMES
[INDEXED BY index-name]
...
- table-name - specifies the table name.
- variable - specifies the data item name.
- integer1 - The number of times the data item should be repeated.
- INDEXED BY index-name - This defines an index for the table. The index can be used to reference specific occurrences within the table.
Single Dimensional Table –
OCCURS clause is used only once to declare a single dimensional table.
Example - Let us declare a table to store two student details. WS-CLASS is the group variable and WS-STUDENT is a variable with all student information OCCURS 2 times to capture the two students information.
---+----2----+----3----+----4----+----5
...
WORKING-STORAGE SECTION.
* Single Dimensional table
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES.
05 WS-ROLL-NO PIC X(03) VALUE "001".
05 WS-NAME PIC X(10) VALUE "STUDENT1".
...
PROCEDURE DIVISION.
DISPLAY "CLASS INFO: " WS-CLASS.
...
JCL to execute the above COBOL program −
//MATEPKRJ JOB MSGLEVEL=(1,1),NOTIFY=&SYSUID //* //STEP01 EXEC PGM=SINDIMTB //STEPLIB DD DSN=MATEPK.COBOL.LOADLIB,DISP=SHR //SYSOUT DD SYSOUT=*
When the program compiled and executed, it gives the following result −
CLASS INFO: 001STUDENT1 001STUDENT1
Two Dimensional Table –
OCCURS clause is used within OCCURS to declare two-dimensional table.
Example - Let us declare a table to store two student details with 6 subjects marks. WS-STUDENT is variable with all student information OCCURS 2 times to capture the two students information. WS-MARKS-GRP is variable that OCCURS 6 times to capture 6 subjects marks.
---+----2----+----3----+----4----+----5
...
WORKING-STORAGE SECTION.
* Two dimensional table
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES.
05 WS-ROLL-NO PIC X(03) VALUE "001".
05 WS-NAME PIC X(10) VALUE "STUDENT1".
05 WS-MARKS-GRP OCCURS 6 TIMES.
10 WS-MARKS PIC 9(03) VALUE 077.
...
PROCEDURE DIVISION.
DISPLAY "CLASS INFO: " WS-CLASS.
...
JCL to execute the above COBOL program −
//MATEPKRJ JOB MSGLEVEL=(1,1),NOTIFY=&SYSUID //* //STEP01 EXEC PGM=TWNDIMTB //STEPLIB DD DSN=MATEPK.COBOL.LOADLIB,DISP=SHR //SYSOUT DD SYSOUT=*
When the program compiled and executed, it gives the following result −
CLASS INFO: 001STUDENT1 077077077077077077001STUDENT1 077077077077077077
Topic | Description |
---|---|
SUBSCRIPT Detailed Tutorial | Table data can be accessed by using subscript. Subscript represents the number of table occurrences. It is automatically created with the OCCURS clause. |
INDEX Detailed Tutorial | Table data can be accessed by using index. Index refers the table element as the number of displacement positions from the table starting position. It is always declared with table and initialized, increment or decrement by SET statement. |
SET Detailed Tutorial | SET statement is used to initialize the table indexes and increasing or decreasing table indexes. |
SEARCH Detailed Tutorial | SEARCH is used to perform a linear search on the table (also known as an array) for a specific item. It works with tables that have the OCCURS clause and are INDEXED BY an index. This SEARCH is called sequential search, serial search, linear search, or simple search. |
SEARCH ALL Detailed Tutorial | SEARCH ALL statement is used to perform binary search on tables (or arrays). It is more efficient for large tables, provided that the table should be in sorted order (either ascending or descending) before the binary search (SEARCH ALL) applies. |
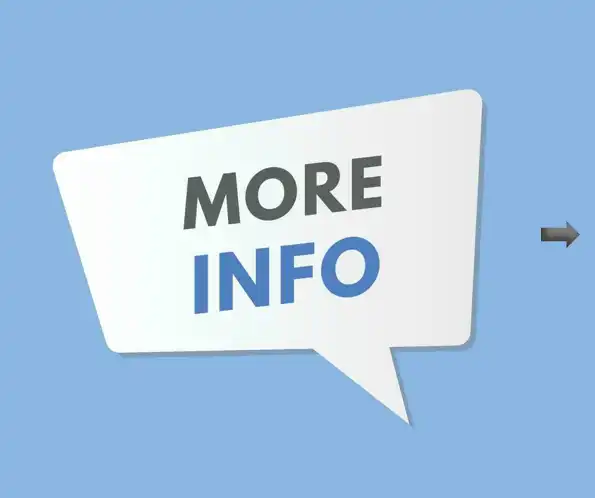