Input Output Statements
Input-output statements are used to manage data transfer between the program's internal storage and external input or output devices. These statements enable functions like reading input or writing output at the runtime.
The input-output statements are –
- ACCEPT Statement
- DISPLAY Statement
ACCEPT Statement
ACCEPT statement receives the data from external sources like JCL or the system during the program execution. ACCEPT places the received information into the variable coded with it.
Syntax -
ACCEPT variable1.
ACCEPT variable2 [FROM DATE [YYYYMMDD]]
[DAY [YYYYDDD]]
[DAY-OF-WEEK]
[TIME].
- variable1 - The receiving variable, which can be an alphanumeric group variable or an elementary variable.
- Variable2 - Specifies the receiving variable.
- DATE - Returns the system date in YYMMDD format. DATE YYYYMMDD returns the system date in YYYYMMDD format.
- DAY - Returns the system date in the format YYDDD. DAY YYYYDDD returns the system date in the format YYYYDDD.
- DAY-OF-WEEK - Returns the system day of the week.
- TIME - Returns the current system time in the format HHMMSSTT.
Example -
Scenario - Receiving data from JCL and receiving the system date using ACCEPT statement.
----+----1----+----2----+----3----+----4----+----5----+
IDENTIFICATION DIVISION.
PROGRAM-ID. ACCPTST.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-VAR.
05 WS-NAME PIC X(20).
05 WS-TODAY PIC 9(08).
PROCEDURE DIVISION.
* Receiving name from JCL
ACCEPT WS-NAME.
* Receiving date from SYSTEM
ACCEPT WS-TODAY FROM DATE YYYYMMDD.
DISPLAY 'RECEIVED NAME: ' WS-NAME.
DISPLAY 'RECEIVED DATE: ' WS-TODAY.
STOP RUN.
JCL -
//MATEPKRJ JOB MSGLEVEL=(1,1),NOTIFY=&SYSUID //** //STEP01 EXEC PGM=ACCPTST //STEPLIB DD DSN=MATEPK.COBOL.LOADLIB,DISP=SHR //SYSIN DD * PAWAN KUMAR Y S /* //SYSOUT DD SYSOUT=*
Output -
RECEIVED NAME: PAWAN KUMAR Y S RECEIVED DATE: 20230706
Explaining Example -
In the above example:
- WS-NAME received from the Run JCL SYSIN DD statement as it has no FROM clause.
- WS-TODAY is obtained from the system in the format YYYYMMDD and requires no input from Run JCL.
DISPLAY Statement
The DISPLAY statement is used to print the variable contents to the output device. The contents are displayed from left to right on the output device.
Syntax -
DISPLAY [variable-name|literal]
- variable-name - refers the variable name.
- literal - refers to the literal coded with double or single quotes.
Examples -
Scenario1 - Basic DISPLAY
WORKING-STORAGE SECTION.
01 WS-VAR.
05 WS-VAR1 PIC X(05) VALUE 'HELLO'.
05 WS-VAR2 PIC X(05) VALUE 'WORLD'.
PROCEDURE DIVISION.
* Simple DISPLAY
DISPLAY "MESSAGE1: " WS-VAR1.
DISPLAY "MESSAGE2: " WS-VAR2.
Output -
MESSAGE1: HELLO MESSAGE2: WORLD
Scenario2 - DISPLAY with multiple items
WORKING-STORAGE SECTION.
01 WS-VAR.
05 WS-VAR1 PIC X(05) VALUE 'HELLO'.
05 WS-VAR2 PIC X(05) VALUE 'WORLD'.
PROCEDURE DIVISION.
* Simple DISPLAY
DISPLAY "MESSAGE: " WS-VAR1 " " WS-VAR2.
Output -
MESSAGE: HELLO WORLD
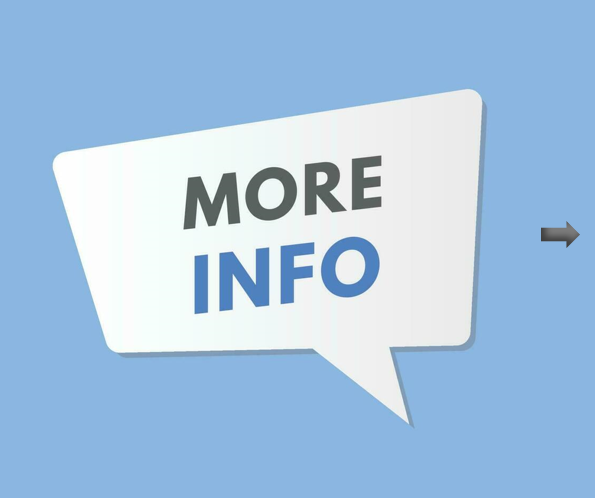