COBOL Internal Sort
Many applications require sorting data in a file or combining multiple files into a single file. Sorting is a method of arranging the given records in either ascending or descending order.
There are two techniques in COBOL to sort the files -
- External sort - DFSORT (SORT) utility in JCL is used to sort files. DFSORT utility is well explained in the Utilities section.
- Internal sort - An internal sort is a method to sort file directly within the program without relying on external utilities. During the sorting process, COBOL reads the records, sorts them in the specified order, and then writes the sorted records either to a new file or back to a file.
Below statements are used in Internal sort process -
Statement | Description |
---|---|
SORT Statement Detailed Tutorial | It is a powerful statement used for sorting files internally within the program. It's mainly useful in batch processing, where large amounts of data need to be sorted before further processing. |
MERGE Statement Detailed Tutorial | It is used to combine two or more sequentially ordered files into a single, merged, and sequentially ordered output file. The files should be in sorted order according to the ascending or descending key that is common for both files. |
RELEASE Statement Detailed Tutorial | It is used to send records from the input procedure to a sort work file in the sorting process. It is only used inside the INPUT PROCEDURE of a SORT operation. |
RETURN Statement Detailed Tutorial | It transfers records from the final phase of a sorting or merging operation to an OUTPUT PROCEDURE. It is used only within the OUTPUT PROCEDURE associated with a SORT or MERGE statement. |
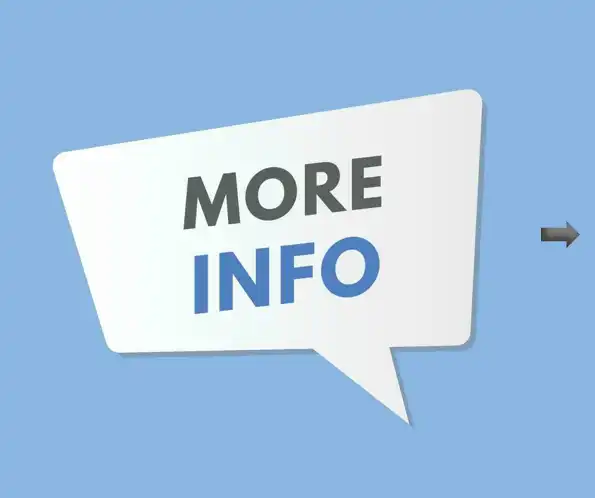