PROCEDURE DIVISION
The PROCEDURE DIVISION is one of the four main divisions of a COBOL program. It's where the program's logic is written and is responsible for carrying out the data processing tasks coded in the program.
The PROCEDURE DIVISION consists of sections, paragraphs, sentences, and statements, which are the actual logic or operations to complete the task. Program execution starts from PROCEDURE DIVISION and ends with STOP RUN or GO BACK statements.
Syntax -
Main program -
PROCEDURE DIVISION.
[section-name SECTION.]
paragraph-name.
[statements...]
Sub program -
PROCEDURE DIVISION [USING {variable-1, variable-2, ...}
RETURNING {variable-a, variable-b, ...}].
[section-name SECTION.]
paragraph-name.
[statements...]
With declaratives -
PROCEDURE DIVISION.
DECLARATIVES.
-----------
-----------
END DECLARATIVES.
- All statements coded in [ ] are optional.
- The PROCEDURE DIVISION begins in Area A and all its statements begins in Area B.
Parameters -
- USING variable-1, variable-2, ... - Optional and specifies when a subprogram is receiving data from main program. The corresponding variables should declare in the LINKAGE SECTION of the subprogram.
- RETURNING variable-a, variable-b, ... - Specifies to receive the result from the subprogram to the main program. It is only applicable to the main(calling) program.
- section-name SECTION - Optional. The PROCEDURE DIVISION can be broken down into sections, which are collections of paragraphs. A section is named and followed by the keyword SECTION.
- paragraph-name - Represents a label for a COBOL statements block. Paragraphs mainly used to organize the logic in the PROCEDURE DIVISION.
- statements - Formed by COBOL keywords to perform an operation.
Practical Example -
Scenario - PROCEDURE DIVISION usage in both main program and subprogram.
Main Program -
----+----1----+----2----+----3----+----4----+----5----+
IDENTIFICATION DIVISION.
PROGRAM-ID. MAINPROG.
AUTHOR. MTH.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-VAR.
05 WS-IP1 PIC 9(02).
05 WS-IP2 PIC 9(02).
05 WS-RESULT PIC 9(04).
05 WS-CALLING-PROG PIC X(08) VALUE "SUBPROG".
PROCEDURE DIVISION.
ACCEPT WS-IP1.
ACCEPT WS-IP2.
CALL WS-CALLING-PROG USING WS-IP1, WS-IP2, WS-RESULT.
DISPLAY "INPUTS: " WS-IP1 ", " WS-IP2.
DISPLAY "RESULTS: " WS-RESULT.
STOP RUN.
SubProgram -
----+----1----+----2----+----3----+----4----+----5----+
IDENTIFICATION DIVISION.
PROGRAM-ID. SUBPROG.
AUTHOR. MTH.
DATA DIVISION.
LINKAGE SECTION.
01 LN-IP1 PIC 9(02).
01 LN-IP2 PIC 9(02).
01 LN-RESULT PIC 9(04).
PROCEDURE DIVISION USING
LN-IP1, LN-IP2 RETURNING LN-RESULT.
COMPUTE LN-RESULT = LN-IP1 * LN-IP2.
STOP RUN.
JCL -
//MATEPKRJ JOB MSGLEVEL=(1,1),NOTIFY=&SYSUID //* //STEP01 EXEC PGM=MAINPROG //STEPLIB DD DSN=MATEPK.COBOL.LOADLIB,DISP=SHR //SYSOUT DD SYSOUT=* //SYSIN DD * 47 25 /*
Output -
INPUTS: 47, 25 RESULTS: 1175
Explaining Example -
- In the above example, MAINPROG is the main program and SUBPROG is the subprogram. Note that USING clause with PROCEDURE DIVISION can use in subprogram only.
- WS-IP1, WS-IP2 are the inputs passed from MAINPROG to the SUBPROG. SUBPROG receives the data into LN-IP1, LN-IP2 from MAINPROG, multiply those values and place the result into LN-RESULT.
- SUBPROG returns the output LN-RESULT to MAINPROG, MAINPROG displays the result received from SUBPROG.
PROCEDURE DIVISION With declaratives -
Declarative is used to code one or more special-purpose sections executed when an exceptional condition occurs. The declarative should be the first section immediately after PROCEDURE DIVISION and should end with the END DECLARATIVES.
Syntax -
PROCEDURE DIVISION.
DECLARATIVES.
-----------
-----------
END DECLARATIVES.
Practical Example -
Scenario - Below example describes how the declaratives use in the COBOL program.
Code -
----+----1----+----2----+----3----+----4----+----5----+
IDENTIFICATION DIVISION.
PROGRAM-ID. PRDECLAR.
AUTHOR. MTH.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-VARS.
05 WS-VAR1 PIC 9(02) VALUE 22.
05 WS-VAR2 PIC 9(02) VALUE 47.
05 WS-MRESULT PIC 9(04).
05 WS-ARESULT PIC 9(04).
PROCEDURE DIVISION.
DECLARATIVES.
DEBUG-DECLARATIVES SECTION.
USE FOR DEBUGGING ON ALL PROCEDURES.
DEBUG-DECLARATIVES-PARAGRAPH.
DISPLAY "TRACE FOR PROCEDURE-NAME : " DEBUG-NAME.
END DECLARATIVES.
MAIN-PROCESSING.
PERFORM PARA-ADDITION.
PERFORM PARA-MULTIPLY.
STOP RUN.
PARA-ADDITION.
COMPUTE WS-ARESULT = WS-VAR1 + WS-VAR2.
DISPLAY "ADDITION RESULT: " WS-ARESULT.
PARA-ADDITION-EXIT.
EXIT.
PARA-MULTIPLY.
COMPUTE WS-MRESULT = WS-VAR1 * WS-VAR2.
DISPLAY "MULTIPLICATION RESULT: " WS-MRESULT.
PARA-MULTIPLY-EXIT.
EXIT.
Compile JCL -
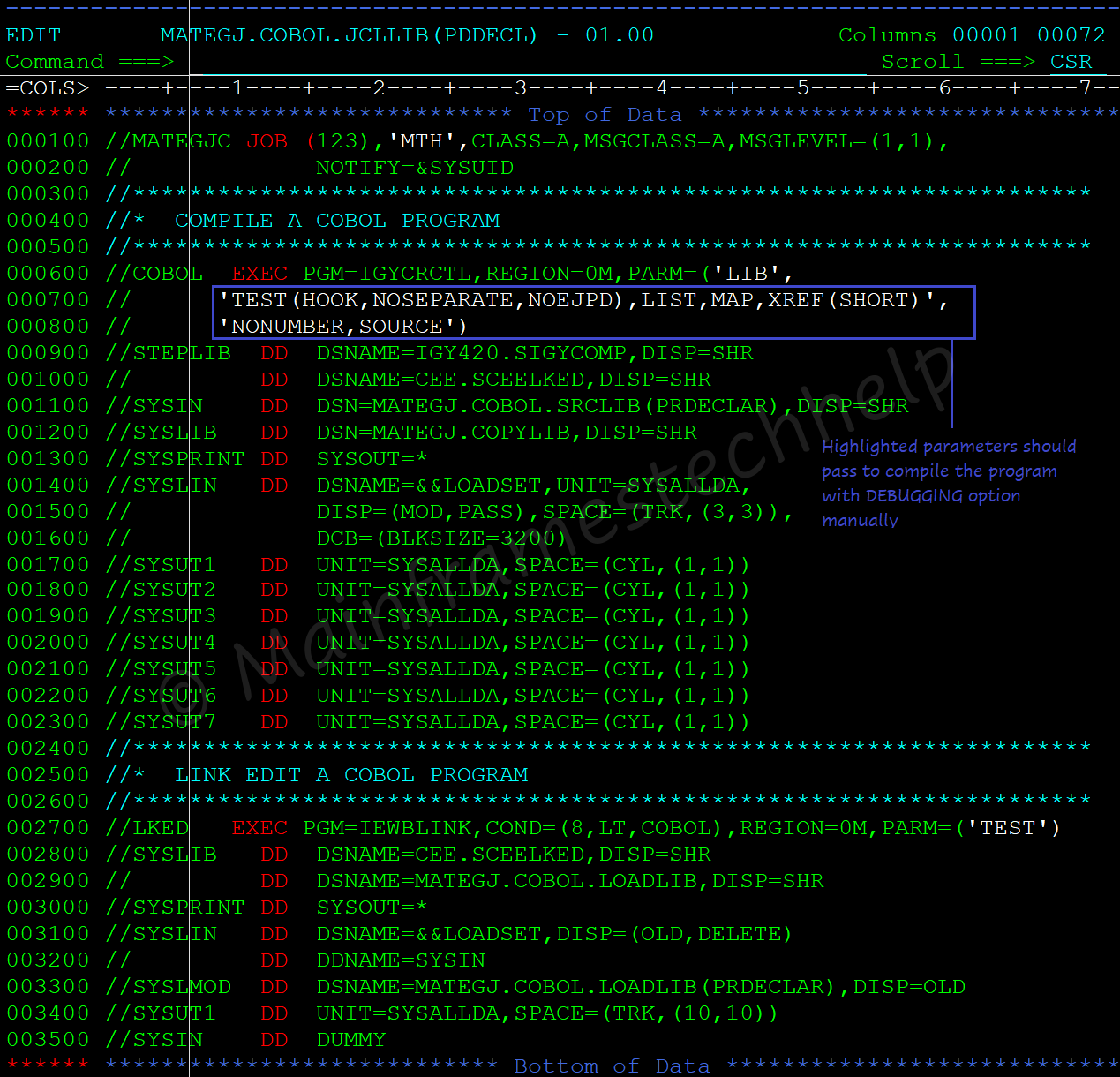
Explaining Example -
To enable the program debugging, the program should compile with TEST (TEST(HOOK,NOSEPARATE,NOEJPD)) option. Once the program is compiled successfully, program trace stat displaying in the output.