COBOL Data Movement Statements
Data movement statements are responsible for transferring data between data items. These are –
- INITIALIZE Statement
- MOVE Statement
INITIALIZE Statement
INITIALIZE sets all elementary variables under group variable with system-defined initial values based on their data types. It is convenient to reset the full copybook and group variable to ensure they start with predictable values.
Syntax -
INITIALIZE group-variable-1 [group-variable-2 ...]
- group-variable-1, group-variable-2, ...- Refers to the variable names we wish to initialize.
Example - Initialize usage in COBOL program.
----+----1----+----2----+----3----+----4----+----5----+
...
WORKING-STORAGE SECTION.
01 WS-VAR.
05 WS-NAME PIC X(20).
05 FILLER PIC X(05).
05 WS-GENDER PIC X(01).
05 FILLER PIC X(05).
05 WS-TODAY PIC 9(08).
05 FILLER PIC X(01).
...
PROCEDURE DIVISION.
MOVE ALL '-' TO WS-VAR.
DISPLAY 'BEFORE INIT: ' WS-VAR.
INITIALIZE WS-VAR.
DISPLAY 'AFTER INIT: ' WS-VAR.
...
JCL -
//MATEPKRJ JOB MSGLEVEL=(1,1),NOTIFY=&SYSUID //** //STEP01 EXEC PGM=INITGRP //STEPLIB DD DSN=MATEPK.COBOL.LOADLIB,DISP=SHR //SYSOUT DD SYSOUT=*
Output -
BEFORE INIT: ---------------------------------------- AFTER INIT: ----- -----00000000-
MOVE Statement
MOVE statement is used to transfer data from the source data item to the target data item. It ensures that data is transformed appropriately or converted based on the receiving data item's type and format.
It is divided into four types based on its usage -
- Simple MOVE
- Group MOVE
- MOVE Corresponding
- MOVE Reference Modification
Simple MOVE
It is used to move the value of the sending item(s) to the receiving item. The sending and receiving data items should be declared with compatible data type to avoid data transferring errors.
Syntax -
MOVE sending-item TO receiving-item.
Example - Moving the alpha-numeric string to another variable.
----+----1----+----2----+----3----+----4----+----5----+
...
WORKING-STORAGE SECTION.
01 WS-SENDING-VAR PIC X(5) VALUE 'Hello'.
01 WS-RECEIVING-VAR PIC X(10).
...
PROCEDURE DIVISION.
MOVE WS-SENDING-VAR TO WS-RECEIVING-VAR.
DISPLAY "WS-RECEIVING-VAR: " WS-RECEIVING-VAR.
...
After the MOVE, WS-RECEIVING-VAR will contain 'Hello' followed by 5 spaces.
Group MOVE
It is used to move data between two groups whose elementary items are in the same order. However, the elementary items in both groups should have the same names and data type.
Syntax -
MOVE sending-group TO receiving-group
Example - Moving data from one group to another group.
----+----1----+----2----+----3----+----4----+----5----+
...
WORKING-STORAGE SECTION.
01 WS-GRP1.
05 NUM PIC 9(02) VALUE 1.
05 NAME PIC X(20) VALUE 'MAINFRAMESTECHHELP'.
01 WS-GRP2.
05 NUM PIC 9(02).
05 NAME PIC X(20).
...
PROCEDURE DIVISION.
MOVE WS-GRP1 TO WS-GRP2.
...
After the MOVE, WS-GRP2 will contain 01MAINFRAMESTECHHELP.
MOVE Corresponding
It is used to move data between two groups whose elementary items are not in the same order. However, the elementary items in both groups should have the same names and data type.
Syntax -
MOVE CORRESPONDING|CORR sending-group TO receiving-group
Example - Changing the date format from MM-DD-YYYY to DD/MM/YYYY.
----+----1----+----2----+----3----+----4----+----5----+
...
WORKING-STORAGE SECTION.
01 WS-DATE-MDY.
05 WS-DATE-MM PIC 9(02) VALUE 10.
05 FILLER PIC X VALUE '-'.
05 WS-DATE-DD PIC 9(02) VALUE 13.
05 FILLER PIC X VALUE '-'.
05 WS-DATE-YYYY PIC 9(04) VALUE 2023.
01 WS-DATE-DMY.
05 WS-DATE-DD PIC 9(02).
05 FILLER PIC X VALUE '/'.
05 WS-DATE-MM PIC 9(02).
05 FILLER PIC X VALUE '/'.
05 WS-DATE-YYYY PIC 9(04).
...
PROCEDURE DIVISION.
MOVE CORR WS-DATE-MDY TO WS-DATE-DMY.
...
After the MOVE, WS-DATE-DMY will contain 13/10/2023.
MOVE Referencing Modification
It is a special-purpose MOVE that moves a portion of data from the sending item to the receiving item. It is mainly useful when dealing with strings, where we might want to handle only a specific part of the data.
Syntax -
variable (start-position [: length])
Example - Formatting full phone number from three different sources.
----+----1----+----2----+----3----+----4----+----5----+
...
WORKING-STORAGE SECTION.
01 WS-COUNTRY-CODE PIC 9(02) VALUE 91.
01 WS-AREA-CODE PIC 9(03) VALUE 999.
01 WS-PHONE-NBR PIC 9(08) VALUE 87654321.
01 WS-FULL-PHN-NBR PIC 9(13).
...
PROCEDURE DIVISION.
MOVE WS-COUNTRY-CODE TO WS-FULL-PHN-NBR(1:2).
MOVE WS-AREA-CODE TO WS-FULL-PHN-NBR(3:2).
MOVE WS-PHONE-NBR TO WS-FULL-PHN-NBR(5:8).
...
After the MOVE, WS-FULL-PHN-NBR will contain 919987654321.
MOVE Combinations -
This table represents the souce item data types(left) and target item data types(top). Y = Move is valid, N = Move is invalid.
Alphabetic | Alpha-numeric | Numeric | Floating-point | |
---|---|---|---|---|
Alphabetic and SPACE | Y | Y | N | N |
Alphanumeric | Y | Y | Y | Y |
Numeric integer and ZERO | N | Y | Y | Y |
Floating-point | N | N | Y | Y |
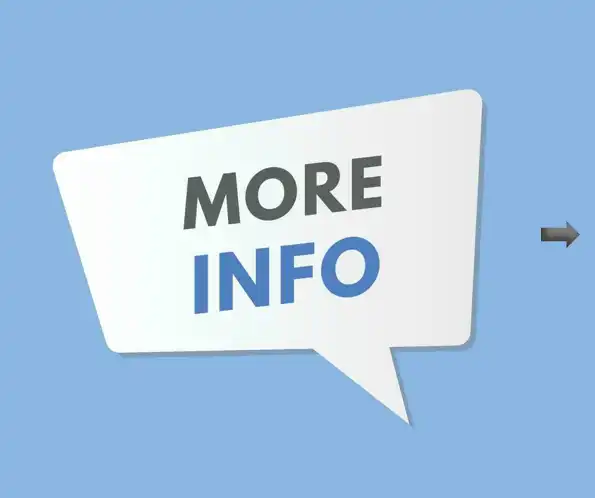