COBOL Arithmetic Statements
COBOL has dedicated statements to perform Arithmetic operations instead of using inline operators like other programming languages. These statements allow developers to perform addition, subtraction, multiplication, division, and more complex computations.
The arithmetic statements in COBOL are -
Statement | Description |
---|---|
ADD Statement Detailed Tutorial | ADD statement performs addition operations. |
SUBTRACT Statement Detailed Tutorial | SUBTRACT statement performs subtract operations. |
MULTIPLY Statement Detailed Tutorial | MULTIPLY statement performs multiplication operations. |
DIVIDE Statement Detailed Tutorial | DIVIDE statement performs division operations. It's similar to mathematics, where we have a dividend (the number being divided) and a divisor (the number we're dividing by). |
COMPUTE Statement Detailed Tutorial | COMPUTE is used to perform all kind of arithmetic operations in a single expression. The arithmetic operators used in COMPUTE statements are + (ADD), - (SUBTRACT), * (MULTIPLY), / (DIVIDE), and ** (EXPONENT). |
Note! The result is stored in the variables associated with GIVING phrase.
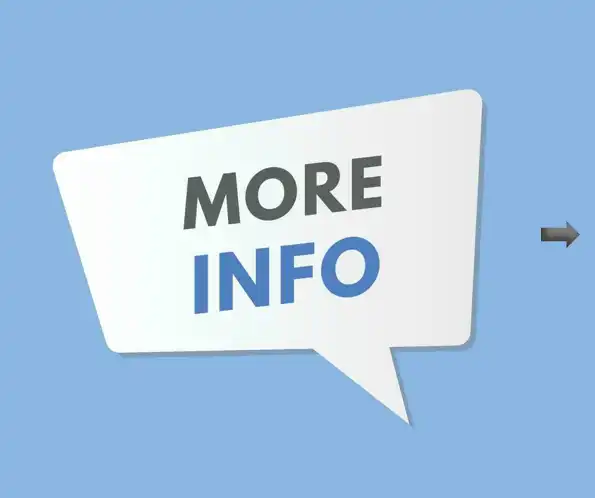