COBOL Program Communication Statements
Program communication statements manage interactions between program components, different programs, or external entities. They enable structured programming by sharing code and data among different parts of a program or between separate programs.
The program communication statements are –
Statement | Description |
---|---|
CALL Statement Detailed Tutorial | It is used to invoke a subprogram to complete a task. The CALL statement is always coded in the calling or main program and the program name in the CALL statement is called or subprogram. |
CANCEL Statement Detailed Tutorial | It is used to release the resources associated with a previously called subprogram. If the subprogram is no longer needed, it is used to free up the resources used by the subprogram. |
STOP RUN Detailed Tutorial | It is used to terminate the execution of a program. Once it is executed, control returns to the operating system. It always uses the main or calling program. |
EXIT PROGRAM Detailed Tutorial | It marks the end of a subprogram (called program) processing and returns control to the main program (calling program). It's mainly used in subprograms, or called programs within COBOL. |
GOBACK Detailed Tutorial | It defines the logical end of a program and gives the control back from where it was received. It can code in both the main program and subprogram. |
GO TO Detailed Tutorial | GO TO transfers control to another part of the program, allowing the program to "jump" to a different paragraph or section. |
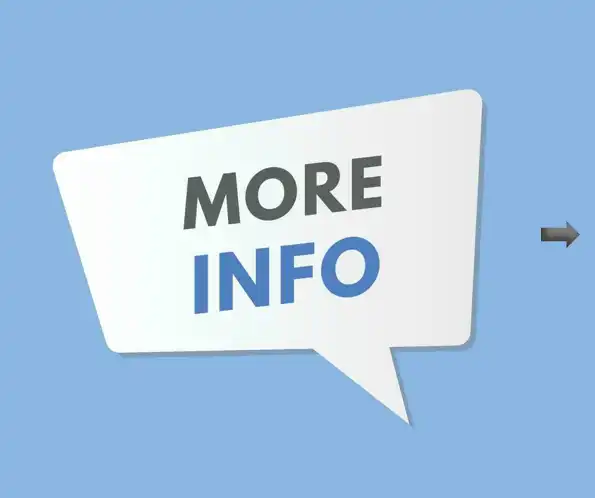