Compiler-directing Statements
- Compiler-directing Statements (often called "directives") provide instructions to the COBOL compiler about how the program should compile and produce the desired object code.
- It's important to note that these directives are not executable code. Instead, they influence the behavior of the compiler during the compilation process.
The critical statements used in the COBOL program are -
COPY Statement
COPY statement includes predefined copybooks (usually file record structures) from the library that is outside of the program.
COPY copybook-name
Example - Insert a copybook into the program.
Copybook - MATEPK.COBOL.COPYLIB(EMPREC)
----+----1----+----2----+----3----+----4----+----5----
05 EMP-NUM PIC 9(05).
05 EMP-NAME PIC X(10).
05 EMP-DESG PIC X(15).
05 EMP-SALARY PIC 9(10).
Code -
----+----1----+----2----+----3----+----4----+----5----
01 EMP-REC.
COPY EMPREC.
Listing after compilation -
----+----1----+----2----+----3----+----4----+----5----
01 EMP-REC.
* COPY EMPREC.
05 EMP-NUM PIC 9(05).
05 EMP-NAME PIC X(10).
05 EMP-DESG PIC X(15).
05 EMP-SALARY PIC 9(10).
EXIT Statement
EXIT statement is a "do-nothing" statement mainly used for readability and structure, giving a logical endpoint for a section or paragraph.
EXIT
Example - EXIT usage in paragraph.
...
PROCEDURE DIVISION.
PERFORM 1000-DISPLAY
THRU 1000-EXIT.
STOP RUN.
1000-DISPLAY.
DISPLAY "PARAGRAPH EXIT EXAMPLE".
1000-EXIT.
EXIT.
Explaining Example -
1000-EXIT is the paragraph coded only with an EXIT statement that acts as an exit paragraph for 1000-DISPLAY.
EJECT Statement
EJECT statement specifies that the next source statement after it should be printed as the first statement of the next page while printing.
EJECT
Example - Coding EJECT statement in COBOL program.
Copybook - MATEPK.COBOL.COPYLIB(EMPREC)
----+----1----+----2----+----3----+----4----+
05 EMP-NUM PIC 9(05).
05 EMP-NAME PIC X(10).
05 EMP-DESG PIC X(15).
05 EMP-SALARY PIC 9(10).
Code -
----+----1----+----2----+----3----+----4----+
01 EMP-REC.
COPY EMPREC.
EJECT.
01 WS-VAR.
05 WS-INPUT1 PIC X(10).
Explaining Example -
The EJECT statement won't display in the program listing. It skips the current page listing, and WS-VAR starts displaying as the first line of the following page listing.
SKIP Statement
SKIP1, SKIP2, or SKIP3 statements specify the number of blank lines that should replace the SKIP statement while listing the COBOL source code.
SKIP1
SKIP2
SKIP3
Example - Coding SKIP1 statement in COBOL program.
----+----1----+----2----+----3----+----4----+----5----
01 EMP-REC.
COPY EMPREC.
SKIP1.
01 WS-VAR.
05 WS-INPUT1 PIC X(10).
Explaining Example -
SKIP1 statement won't display in the program listing. It inserts one blank line before WS-VAR declaration line in the program listing.
Other Statements -
Statement | Usage |
---|---|
BASIS statement | It is an extended library statement and specifies the complete COBOL program as a source for compilation. |
CALLINTERFACE directive | Specifies the interface for CALL and SET statements. |
DELETE statement | It is an extended library statement that removes COBOL source statements from the BASIS source program. |
ENTER statement | Treats as a comment |
INSERT statement | Library statement adds COBOL statements to the source program. |
PROCESS (CBL) statement | Should code before IDENTIFICATION DIVISION and used to code compiler options that are to be used to compile the module. |
REPLACE statement | Used to replace the source program text. |
SERVICE LABEL statement | Generates by the CICS translator and used to specify the control flow. |
TITLE statement | Specifies the header that should print on top of each page. |
USE statement | Provides the declarative. |
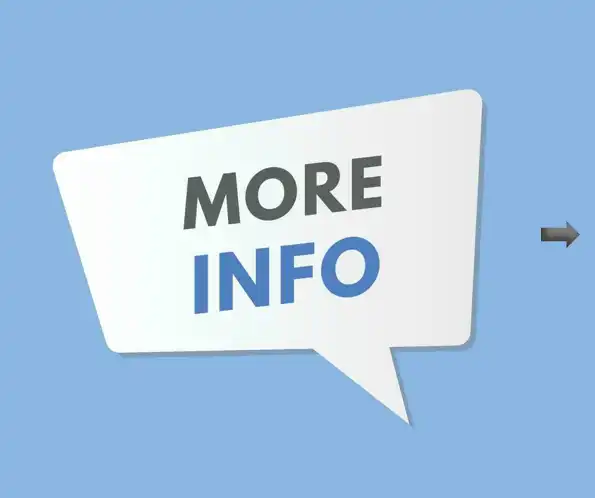