Basic Verbs
Every programming language is designed with some system-defined set of characters, symbols, keywords, and standards. Similarly, COBOL has its own set of characters, symbols, keywords, and standards. Below are the basic and known terms in the COBOL –
- Character set
- Character strings
- COBOL Words
- Variables
- Literals
- Constants
- Figurative Constants
- Comments
- Separators
Character set
The character set refers to the collection of valid characters. They define literals, variables, and other identifiers in a COBOL program.
The COBOL language has its own set of valid characters (78) that contains alphabets (A-Z | a-z), digits (0-9), and special characters. The list of basic COBOL characters is as follows –
Character | Meaning | Character | Meaning |
---|---|---|---|
Space | ' | Apostrophe | |
+ | Plus | ( | Left parenthesis |
- | Minus or hyphen | ) | Right parenthesis |
* | Asterisk | > | Greater than |
/ | Forward slash or solidus | < | Less than |
= | Equal sign | : | Colon |
$ | Currency sign | _ | Underscore |
, | Comma | A - Z | Alphabet (uppercase) |
; | Semicolon | a - z | Alphabet (lowercase) |
. | Decimal point or period | 0 - 9 | Numeric characters |
" | Quotation mark |
Character Strings
The character string is a set of characters created for a purpose or to name something. Character strings creates -
- COBOL Words
- Variables
- Literals
- Constants
- Figurative Constants
- Comments
COBOL Words
A COBOL word is a set of characters, and each character is from the following character set -
- Latin uppercase letters A through Z
- Latin lowercase letters a through z
- digits 0 through 9
- - (hyphen)
- _ (underscore)
COBOL word minimum length is one character, and the maximum is 30 characters.
Rules -
- Each lowercase alphabet is equivalent to its uppercase.
- The hyphen(-) or underscore(_) cannot appear as the first or last character.
COBOL Word Types -
COBOL words are two types, and those are -
- User-defined Words - Any word that is defined by the developer is called as a user-defined word and is used to store the data. It can be of maximum length is 30 bytes (except for level-numbers). They can be anything from MTHPROG1, STD-GENDER, reflecting the unique aspects of your COBOL program. For Example - MTHPROG1, STD-GENDER, ....
- Reserved Words - A reserved word is a system-defined word with proper meaning or task assigned in COBOL language. Reserved words can be - keywords, optional words, figurative constants, special character words and special registers. For Example - ACCEPT, SKIP1, ZEROS, ....
Variable
A Variable is used to store and process the value. It holds a pointer to memory location where actually the value stored. Every variable should be declared in the DATA DIVISION of the COBOL program.
A variable declaration should always contain the data type with its length (except the group variable). A Variable is also called a data item. For Example - WS-A, WS-VAR, WS-TOTAL, WS-INPUT, WS_OUTPUT, etc.
Literal
The literal is the value that is assigned to the variable. The value can be a string or number. Literals are two types –
- Non-numeric literals - Non-numeric literals are the strings enclosed by quotation marks(") or apostrophes(').
It can contain all valid character that are allowed by COBOL.
For Example - "HELLO", "THIS ISN'T WRONG", etc. - Numeric literals - A numeric literal is a number that is a combination of a sign (+ or -), and a decimal point.
A numeric literal codes directly without quotation marks(" ") and apostrophes(' ').
For Example - 1234, -1234, etc.
Constant
A variable is initialized with a literal. If the variable value doesn't change during the program's execution, the variable is considered as a constant variable, and the value is considered as a constant value.
Constant values are three types and those are –
- Numeric constants – Numeric variables having one value throughout the program execution are called numeric constants. 3.14 is numeric constant .
For Example - 01 WS-PI PIC 9(2)V9(2) VALUE 3.14. - Alphanumeric | non-numeric constants – Alphanumeric variables with only one value throughout the program execution are called alphanumeric constants. HI is the non-numeric constant.
For Example - 01 WS-HI PIC X(05) VALUE "HI". - Figurative Constants - System-defined constants are predefined in the COBOL and used as replacements for standard values like spaces, zeroes, etc. ZEROES is the figurative constant.
For Example - 01 WS-VAR PIC 9(5) VALUE ZEROES.
Figurative constants are system-defined keywords with predefined values. The figurative constants in COBOL are -
Figurative constant | Description |
---|---|
ZERO, ZEROS, ZEROES |
Represents one or more occurrences of the numeric value 0. ZERO is a single 0 and ZEROS or ZEROES means two or more occurrences of 0s. |
SPACE, SPACES |
Represents one or more occurrences of the space. i.e., " " or X'40'. SPACE is single SPACE (" "), and SPACES means two or more occurrences of space. |
HIGH-VALUE, HIGH-VALUES |
Represents one or more occurrences of the high-value. i.e., X'FF'. |
LOW-VALUE, LOW-VALUES |
Represents one or more occurrences of the low-value. i.e., X'00'). |
QUOTE, QUOTES |
Represents one or more occurrences of quotation mark (") or apostrophe ('). |
ALL | Represents one or more occurrences of the character string or figurative constant. |
Comments
A comment is a non-executable statement that provides the information about code or business requirements. All computer-supported characters are allowed to write comments, and they do not affect the program's execution.
These are three types based on their usage and where they are used -
- IDENTIFICATION DIVISION Comments - Entries with optional paragraphs in IDENTIFICATION DIVISION are comments and their usage is -
AUTHOR. NameOfProgrammer. INSTALLATION. Development-center. DATE-WRITTEN. mm/dd/yy. DATE-COMPILED. mm/dd/yy. HH:MM:SS. SECURITY. Program-type.
- Full line comments (any division)
Any line starting with an asterisk (*) in column 7 (indicator area) is a full-line comment. Comments can be coded in any division.For example -----+----1----+----2----+----3----+----4----+----5 * FULL LINE COMMENT WITH * IN COLUMN-7.
- Floating comment indicator (*>) -
Comment starts in the middle of any line between 8-72 columns. For Example -01 WS-VAR PIC X(12). *> INLINE COMMENT
Separators
A separator is a single or multiple character that separates words or strings. The table below shows the separators list -
Separator | Meaning | Separator | Meaning |
---|---|---|---|
Space | : | Colon | |
, | Comma | " | Quotation mark |
. | Period | ' | Apostrophe |
; | Semicolon | == | Pseudo-text delimiter |
Practical Example -
Scenario - Below screenshot describes the different types of character strings in COBOL programming.
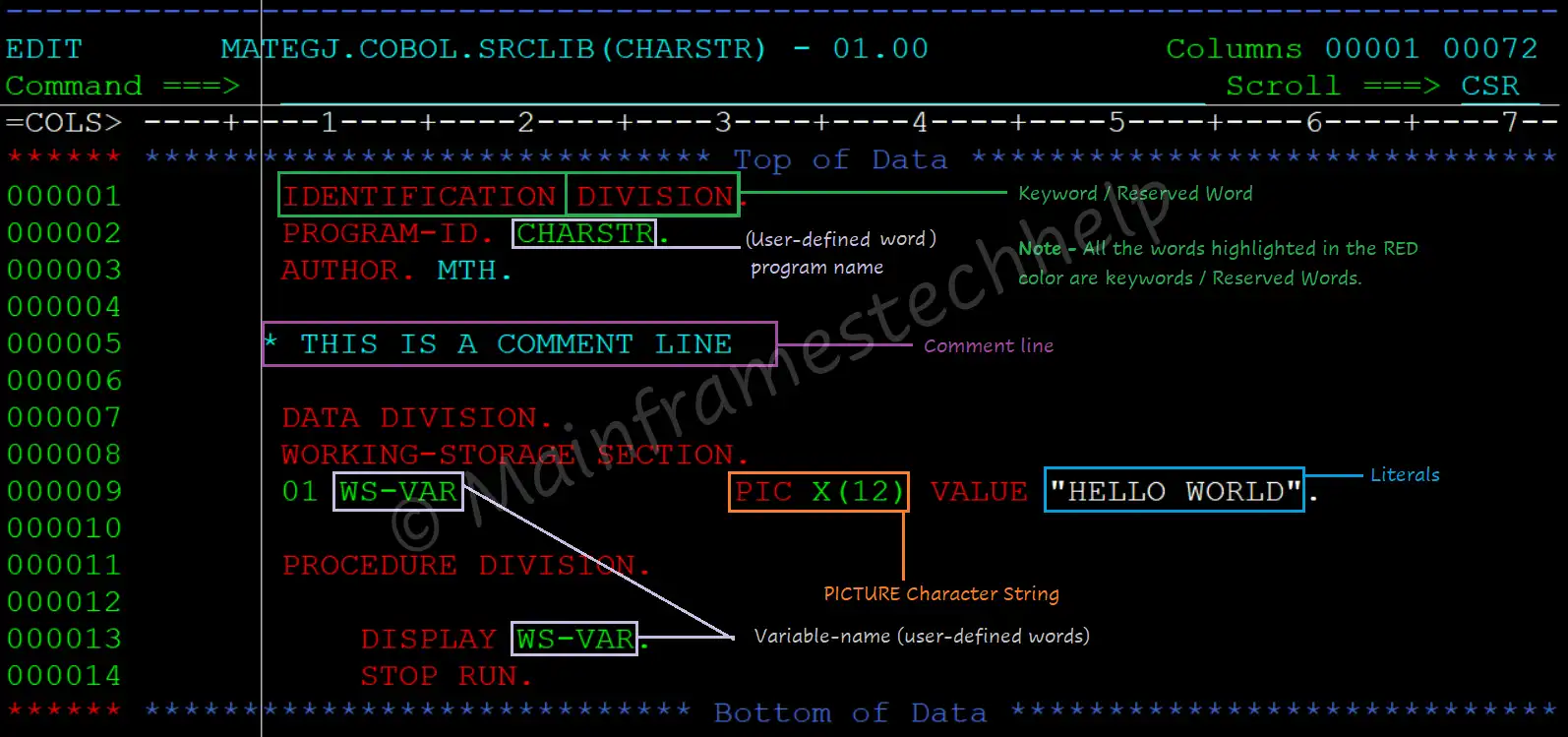
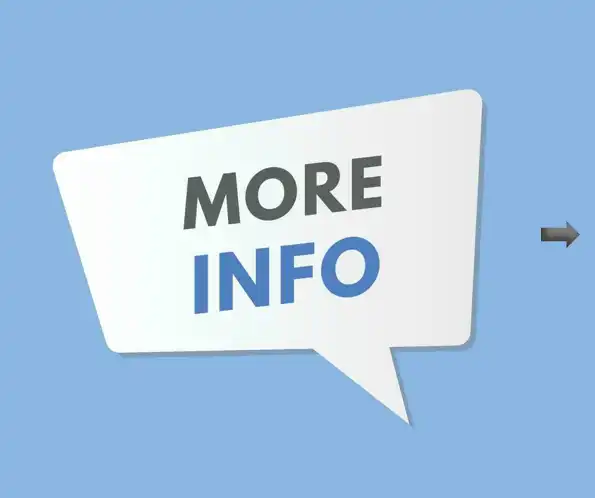