File Organization
File organization is the method used to store and arrange data records within a file. It specifies how information is physically stored in the memory and impacts how efficiently data can be accessed and managed.
COBOL primarily supports three types of file organizations -
- Sequential File Organization
- Indexed File Organization
- Relative File Organization
The selection of file organization is important because it affects how efficiently a program can access and manage data. We will discuss all file organizations below, which will help you select the best organization based on your requirements.
Sequential File Organization -
Sequential file organization applies to the sequential files (PS - Physical sequential file) that use the QSAM access method. A sequential file consists of records that are stored and accessed sequentially. The main attributes of the sequential file organization are -
- Records are stored in the same order as they are written.
- Records can be read in sequential order (entry sequential) from file beginning to end. For example, if we need to read the 5th record, we need to read five times to get the desired record.
- Record physical deletion or length change is not possible once it is written to the file.
- Updating a record (REWRITE) is possible. A record can be updated with new data or replaced with a new record if both have the same length.
- A new record is always written at the end of the file.
- Records sorting is not possible.
- The file may contain duplicate records because the key is not applicable.
- Accessing is slow because reading is allowed only in sequential order.
Syntax -
ENVIRONMENT DIVISION.
INPUT-OUTPUT SECTION.
FILE-CONTROL.
SELECT logical-file-name ASSIGN TO DSName
ORGANIZATION IS SEQUENTIAL
Example - Declaring a sequential file in program.
ENVIRONMENT DIVISION.
INPUT-OUTPUT SECTION.
FILE-CONTROL.
SELECT empfile ASSIGN TO input01
ORGANIZATION IS SEQUENTIAL
Indexed File Organization -
Indexed file organization applies to the KSDS file that uses the Virtual storage access method (VSAM). A KSDS file consists of records that are stored and accessed using the key value. The key is unique and combination of field or fields (defined by the developer and part of the record). VSAM file has two components -
- DATA - Contains the actual data.
- INDEX - Contains the key information along with its pointers to record stored in memory.
The main attributes of the indexed file organization are -
- Records are written in key sequential order.
- Records can be read sequentially (one by one without key value), randomly (using key-value), or dynamically (a combination of the above two).
- Physical deletion of the record is allowed, and the memory released will be reused.
- Updating a record (REWRITE) is possible.
- New records will be written where the key value fits in sorting order.
- All the records in the file are sorted order based on key values.
- Duplicate records cannot be written into the file because of the key.
- Accessing records is faster because we can read them dynamically or randomly.
Syntax -
ENVIRONMENT DIVISION.
INPUT-OUTPUT SECTION.
FILE-CONTROL.
SELECT logical-file-name ASSIGN TO DSName
ORGANIZATION IS INDEXED
RECORD KEY IS primary-key
ALTERNATE KEY IS alt-key
Example - Declaring indexed file in program with key emp-no and alternate key emp-dept.
ENVIRONMENT DIVISION.
INPUT-OUTPUT SECTION.
FILE-CONTROL.
SELECT empfile ASSIGN TO input01
ORGANIZATION IS INDEXED
RECORD KEY IS emp-no
ALTERNATE KEY IS emp-dept
Relative File Organization -
Relative file organization applies to the RRDS file that uses the Virtual storage access method (VSAM). An RRDS file consists of records that are stored and accessed using the RRN value. Relative Record Number (RRN) is unique and used as an implicit key (generated by the system and not part of the record).
The main attributes of the indexed file organization are -
- Records are written in Record Relative Number (RRN) order.
- Records can be read sequentially (one by one without RRN value), randomly (using RRN-value), or dynamically (combination of the above two).
- Physical deletion of the record is allowed, but the memory is not reused.
- Updating a record (REWRITE) is possible.
- New records will be written sequentially and set RRN value for it.
- All the records in the file are sorted order based on RRN.
- Duplicate records can't be written into the file.
- Records accessing is faster than indexed files as the records can be read using RRN.
Syntax -
ENVIRONMENT DIVISION.
INPUT-OUTPUT SECTION.
FILE-CONTROL.
SELECT logical-file-name ASSIGN TO DSName
ORGANIZATION IS RELATIVE
RELATIVE KEY IS rrn
Example - Declaring Relative file in program with key emp-rrn.
ENVIRONMENT DIVISION.
INPUT-OUTPUT SECTION.
FILE-CONTROL.
SELECT empfile ASSIGN TO input01
ORGANIZATION IS RELATIVE
RELATIVE KEY IS emp-rrn
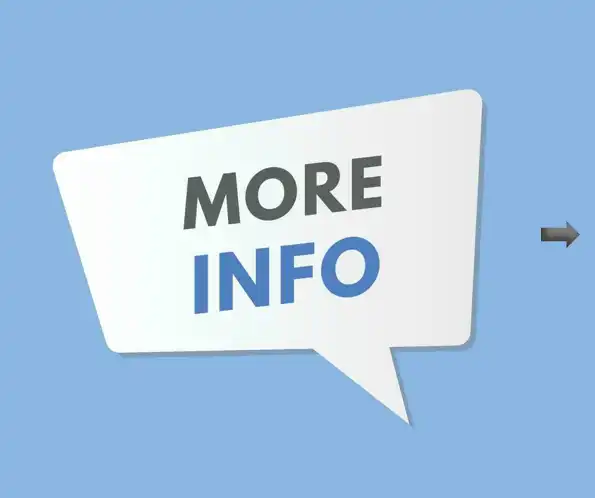