COBOL String Handling Statements
String handling statements are used to handle and manipulate strings. These statements are essential for processing textual data, allowing for modifications, evaluations, and extractions of character data within a COBOL program.
COBOL offers various language options for performing different operations on string data items. For example, we can:
- Join or split strings.
- Manipulate null-terminated strings by counting or moving characters.
- Access substrings by their ordinal position and specify their length if needed.
- Tally and replace strings, such as counting how many times a specific character appears in a data item.
- Convert data items, for instance, by changing them to uppercase or lowercase.
- Evaluate data items, including determining the length of a data item.
String handling statements are -
Statement | Description |
---|---|
STRING Statement Detailed Tutorial | STRING statement concatenates two or more strings or literals into a single string and places them into a result variable. It enables the creation of a single string from multiple stings separated by delimiter. |
UNSTRING Statement Detailed Tutorial | UNSTRING statement takes a single string, breaks it down into several separate strings, and places them into the variables. It breaks the strings into multiple stings based on the delimiter. |
INSPECT Statement Detailed Tutorial | INSPECT statement analyzes, counts, or replaces specific character(s) within a string.
It is flexible and provides a range of functions to help with string manipulations.
It has four formats -
|
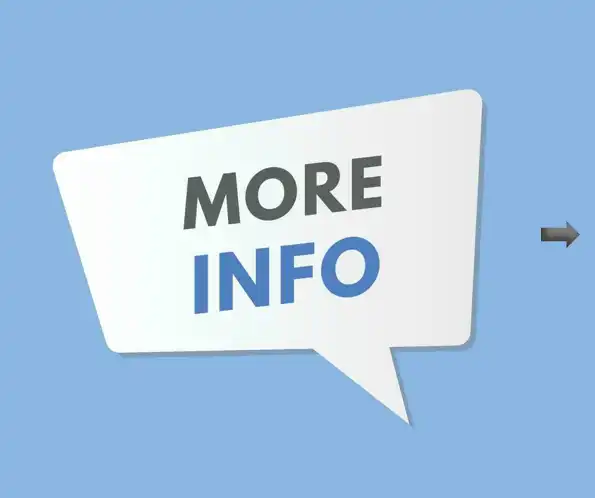