COBOL INSPECT Statement
INSPECT statement analyzes, counts, or replaces specific character(s) within a string. It is flexible and provides a range of functions to help with string manipulations.
INSPECT statement has four formats -
- INSPECT...TALLYING
- INSPECT...REPLACING
- INSPECT...TALLYING...REPLACING
- INSPECT CONVERTING
INSPECT...TALLYING
INSPECT TALLYING counts the occurrences of the specific characters in the input string. It is an efficient way to decide how many times a particular character appears within a string.
INSPECT ws-input-string
TALLYING ws-tally-count
FOR [ALL|LEADING] {CHARACTERS|ws-tally-chars}
- ws-input-string - Specifies the input string or variable.
- ws-tally-count - A numeric variable where the count is stored.
- FOR CHARACTERS - Each character is counted.
- ws-tally-char - Specifies the characters that should find the number of occurrences in the count ws-input-string.
- ALL - Each occurrence of ws-tally-char is counted.
- LEADING - leftmost occurrence of ws-tally-chars is counted.
Examples -
Scenario1 - Counting for ALL character "A".
Input- WS-DATA = "MAINFRAMES" Declaration- 05 WS-DATA PIC X(10) VALUE "MAINFRAMES". 05 WS-CNT PIC 9(02). Code- INSPECT WS-DATA TALLYING WS-CNT FOR ALL "A". Result- WS-CNT = 2
Scenario2 - Counting for characters.
Input- WS-DATA = "MAINFRAMES" Declaration- 05 WS-DATA PIC X(10) VALUE "MAINFRAMES". 05 WS-CNT PIC 9(02). Code- INSPECT WS-DATA TALLYING WS-CNT FOR CHARACTERS. Result- WS-CNT = 10
In the above case, WS-DATA has 10 characters. So the result is 10.
INSPECT...REPLACING
INSPECT...REPLACING replaces the replacing string with the replacing string in the source string. It's a way to replace all instances of certain characters with target characters.
INSPECT ws-input-string
REPLACING [ALL|LEADING|FIRST] {CHARACTERS|ws-replaced-char}
BY ws-replacing-char
- ws-replaced-char - Specifies the characters in string to replace.
- ws-replacing-char - Specifies the characters that replaces ws-replaced-char.
- ALL CHARACTERS - Replace each occurrence of character with a ws-replacing-char.
- LEADING - Replaces leftmost occurrence of ws-replaced-char by ws-replacing-char.
- FIRST - Replaces the leftmost first occurrence of ws-replaced-char by ws-replacing-char.
Examples -
Scenario1 - Replace all "-" with "/".
Input- WS-DATA = "DD-MM-YYYY" Declaration- 05 WS-DATA PIC X(10) VALUE "DD-MM-YYYY". Code- INSPECT WS-DATA REPLACING ALL "-" BY "/". Result- WS-DATA = "DD/MM/YYYY"
Scenario2 - Replace characters with '$'.
Input- WS-DATA = "MAINFRAMES" Declaration- 05 WS-DATA PIC X(10) VALUE "MAINFRAMES". Code- INSPECT WS-DATA REPLACING CHARACTERS BY "$". Result- WS-DATA = "$$$$$$$$$$"
In the above case, every character is replaced by '$'. So the result is "$$$$$$$$$$".
INSPECT...TALLYING...REPLACING
INSPECT TALLYING REPLACING counts the occurrences of the specific characters and replaces them with new characters. It performs the TALLYING operation first and REPLACING next.
INSPECT ws-input-string
TALLYING ws-tally-count
FOR [ALL|LEADING] {CHARACTERS|ws-tally-chars}
REPLACING [ALL|LEADING|FIRST] {CHARACTERS|ws-replaced-char}
BY ws-replacing-char
Examples -
Scenario1 - Count for all "-" and Replace all "-" with "/".
Input - WS-DATA = "DD-MM-YYYY" Declaration - 05 WS-DATA PIC X(10) VALUE "DD-MM-YYYY". 05 WS-CNT PIC 9(02) VALUE ZEROES. Code- INSPECT WS-DATA TALLYING WS-CNT FOR ALL "-" REPLACING ALL "-" BY "/". Result - WS-CNT = 2 WS-DATA = "DD/MM/YYYY"
Scenario2 - Count for no of characters and Replace them with "&".
Input- WS-DATA = "DD-MM-YYYY" Declaration- 05 WS-DATA PIC X(10) VALUE "DD-MM-YYYY". 05 WS-CNT PIC 9(02) VALUE ZEROES. Code- INSPECT WS-DATA TALLYING WS-CNT FOR CHARACTERS REPLACING CHARACTERS BY "&". Result- WS-CNT = 10 WS-DATA = "&&&&&&&&&&"
In the above case, WS-DATA has 10 characters. So the count result is 10 and replaces all characters with "&". The result is "&&&&&&&&&&".
INSPECT...CONVERTING
INSPECT...CONVERTING performs character conversion in a variable. It's a way to convert all instances of certain characters with other characters.
INSPECT ws-input-string
CONVERTING ws-char-1 .... char-n
TO char-a ... char-z
[[BEFORE | AFTER] [INITIAL] ws-delimeter].
- char-1 ... char-n - Specifies the character(s) we're searching for.
- char-a ... char-z - specifies the character(s) that will replace the characters char-1 ... char-n during the conversion.
Examples -
Scenario1 - Conveting all uppercase characters to lowercase.
Input- WS-DATA = "MAINFRAMES" Declaration- 05 WS-DATA PIC X(10) VALUE "MAINFRAMES". Code- INSPECT WS-DATA CONVERTING "ABCDEFGHIJKLMNOPQRSTUVWXYZ" TO "abcdefghijklmnopqrstuvwxyz". Result- WS-DATA = "mainframes"
The first character, 'M', converts to its equal character, 'm'. In the second iteration, the second character, 'A', converts to its equal character, 'a'. Similarly, every character is converted with its equal characters. The result is 'mainframes'.
Scenario2 - Conveting all uppercase characters to lowercase before "R".
Input- WS-DATA = "MAINFRAMES" Declaration- 05 WS-DATA PIC X(10) VALUE "MAINFRAMES". Code- INSPECT WS-DATA CONVERTING "ABCDEFGHIJKLMNOPQRSTUVWXYZ" TO "abcdefghijklmnopqrstuvwxyz" BEFORE "R". Result- WS-DATA = "mainfRAMES"
In the above case, it converts all uppercase characters to lowercase characters before "R".
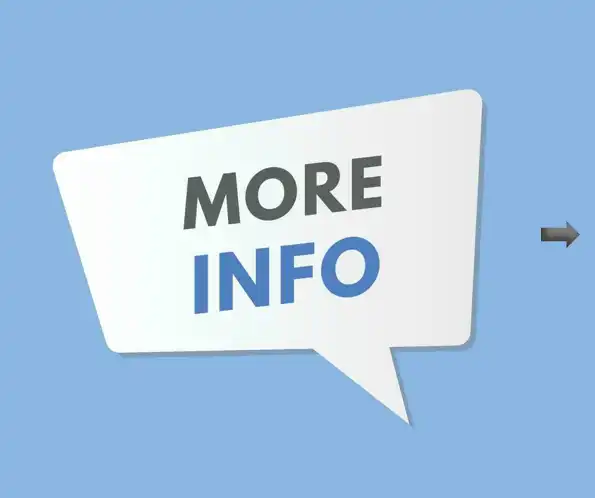