Divisions
A division is one of the primary part of a program, organizing its content and functionality. A division is a collection of one or more sections and paragraphs.
Division begins with the division name and ends at the beginning of the subsequent division or the program ends. All divisions are system-defined and should begin in Area A. COBOL has four system-defined divisions, and those are -
- IDENTIFICATION DIVISION
- ENVIRONMENT DIVISION
- DATA DIVISION
- PROCEDURE DIVISION
IDENTIFICATION DIVISION
The IDENTIFICATION DIVISION is one among the four divisions and should be the first division in COBOL program. It provides metadata about the program, such as its name, author, installation, and other descriptive information used to identify the program uniquely.
Syntax -
----+----1----+----2----+----3----+----4----+----5----+
IDENTIFICATION DIVISION.
PROGRAM-ID. NameOfProgram.
[AUTHOR. NameOfProgrammer.]
[INSTALLATION. Installation-details.]
[DATE-WRITTEN. program-written-date.]
[DATE-COMPILED. program-compiled-date.]
[SECURITY. security-status.]
Parameters -
- PROGRAM-ID - Mandatory. It provides the name of the COBOL program. This name is used by the OS when the program is called or executed.
- AUTHOR (optional) - Provides the name of the individual, team, or organization that wrote the program.
- INSTALLATION (optional) - Offers details about the computer installation where the program was developed or is intended to run.
- DATE-WRITTEN (optional) - Specifies the date when the program was originally written.
- DATE-COMPILED (optional) - Gives the date when the program was last compiled. Some compilers or environments may automatically fill in this entry.
- SECURITY (optional) - Indicates the program's security level or classification. It's typically used in environments with specific security requirements.
Example - Explaing about IDENTIFICATION DIVISION entries.
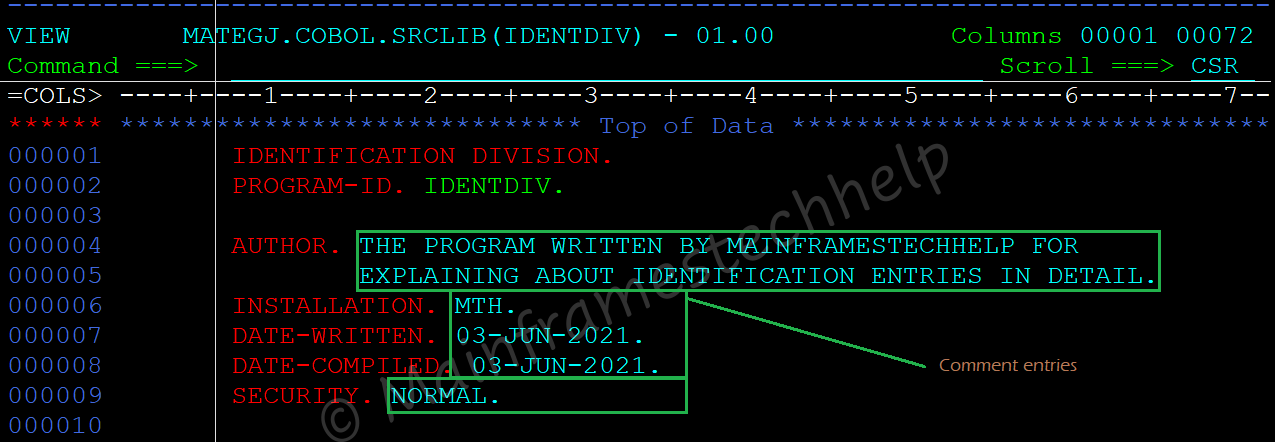
ENVIRONMENT DIVISION
The ENVIRONMENT DIVISION specifies the computer environment in which a program runs. This division is primarily involved with file specifications and provides the interface between the program's data and the outside world (like input and output devices or files). It is divided into two sections: the CONFIGURATION SECTION and the INPUT-OUTPUT SECTION.
Syntax -
ENVIRONMENT DIVISION.
[CONFIGURATION SECTION.
[SOURCE-COMPUTER. source-computer-name [WITH DEBUGGING MODE]
[OBJECT-COMPUTER. object-computer-name]
[SPECIAL-NAMES. special-names-enties]]
[INPUT-OUTPUT SECTION.
[FILE-CONTROL. file-control-entries]
[I-O-CONTROL. i-o-control-entries]]
Parameters -
- SOURCE-COMPUTER - Specifies the computer on which the source program is compiled. WITH DEBUGGING MODE is used to enable the debugging switch in the program.
- OBJECT-COMPUTER - Specifies the computer where the object program is to be executed.
- SPECIAL-NAMES - Specifies system-specific features, such as mapping symbolic characters to system-specific characters or defining currency signs.
- FILE-CONTROL - Used to map logical files (as named in the program) to physical files on the system.
- I-O-CONTROL - Provides specifications for the computer's input-output operations, like multiple file tapes or devices.
Examples - Example to enable DEBUGGING MODE.
ENVIRONMENT DIVISION.
CONFIGURATION SECTION.
SOURCE-COMPUTER. IBM3278 WITH DEBUGGING MODE.
PROCEDURE DIVISION.
D DISPLAY "DISPLAYING DEBUGGING LINE".
DISPLAY "DISPLAYING NORMAL LINE".
Output -
DISPLAYING DEBUGGING LINE DISPLAYING NORMAL LINE
DATA DIVISION
DATA DIVISION defines the variables that are used in the program for data processing. It represents the logical structure of the data, laying out how it is stored in memory. It is optional.
Syntax -
DATA DIVISION.
[FILE SECTION.
FD|SD file-description-entry...
[data-description-entry...]]
[WORKING-STORAGE SECTION.
data-description-entry...]
[LINKAGE SECTION.
data-description-entry...]
Parameters -
- FILE SECTION - This section is used to describe the layout of files that are read from or written to. FD is used for physical files. SD is used for sort files.
- WORKING-STORAGE SECTION - Defines variables and constants that are used throughout the program. These are typically initialized each time the program starts.
- LINKAGE SECTION - Used to describe data that is passed into or out of the program. It's especially useful when working with subprograms or modules.
Examples - Declaring a file with length 80.
----+----1----+----2----+----3----+----4----+
DATA DIVISION.
FILE SECTION.
FD file1
RECORD CONTAINS 80 CHARACTERS
BLOCK CONTAINS 800 CHARACTERS
RECORDING MODE IS F
DATA RECORD IS emp-rec.
FILE STATUS IS ws-fs.
01 emp-rec PIC X(80).
WORKING-STORAGE SECTION.
01 ws-fs PIC 9(02).
PROCEDURE DIVISION
The PROCEDURE DIVISION is where the program's logic is written and is responsible for carrying out the data processing tasks coded in the program. Program execution starts from PROCEDURE DIVISION and ends with STOP RUN or GO BACK statements.
Syntax -
PROCEDURE DIVISION [USING parameter...].
[section-name SECTION.]
paragraph-name.
[statements...]
[additional-paragraphs...]
[... more sections and paragraphs ...]
Parameters -
- USING parameter - It is optional. When a program can be CALLED from another program, we use the USING clause to pass data between them. The parameters correspond to the LINKAGE SECTION entries.
- section-name SECTION - It is optional. The PROCEDURE DIVISION can be organized into sections, each of which can contain one or more paragraphs. A section name ends with the word SECTION.
- paragraph-name - A label that marks a set of related statements.
- statements - The executable COBOL statements that perform operations on data, make decisions, manipulate files, and more.
Examples - PROCEDURE DIVISION with declaratives.
----+----1----+----2----+----3----+----4----+
...
PROCEDURE DIVISION.
DECLARATIVES.
DEBUG-DECLARATIVES SECTION.
USE FOR DEBUGGING ON ALL PROCEDURES.
DEBUG-DECLARATIVES-PARAGRAPH.
DISPLAY "TRACE FOR PROCEDURE-NAME : " DEBUG-NAME.
END DECLARATIVES.
...
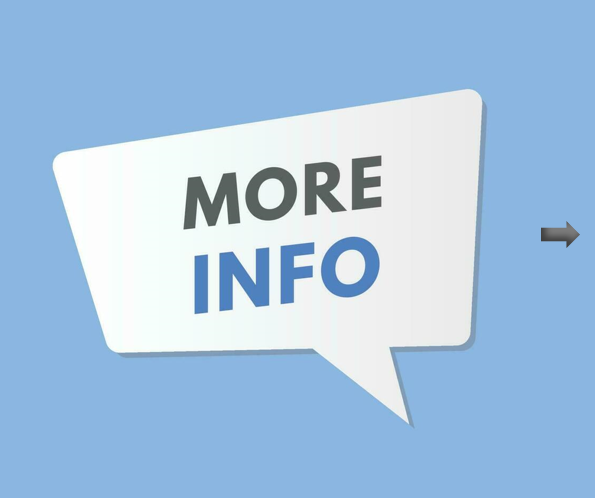